The Real Starman?
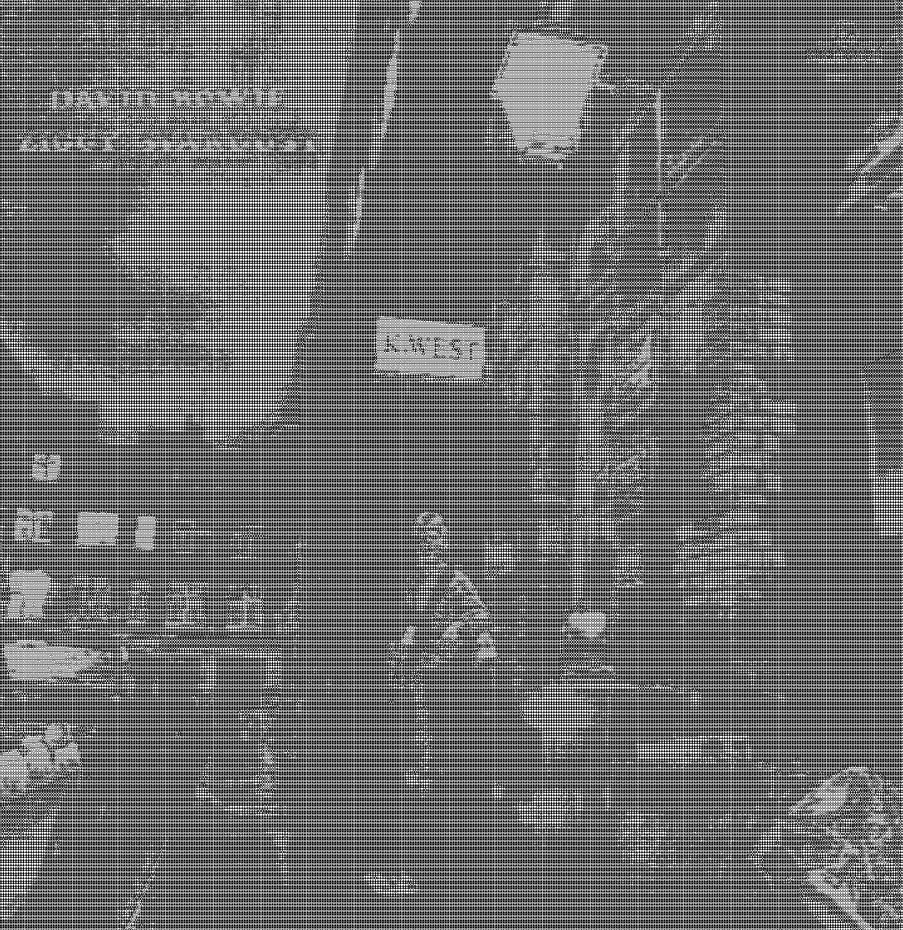
I found a crazy klek hidden in the Ziggy Stardust album cover!
I don’t really know how I went from reading https://smallweb.site/formsites/inspace to writing a Python script to turn images into greyscale images rendered in ascii star characters, but somehow I did!
The script follows a really simple algorithm, going through each 2x2 square of pixels, finding the average luminence of each square, and printing out a different ascii star character based on the luminence.
Scrappy code:
from PIL import Image
import sys
if len(sys.argv) != 2:
raise Exception("Need to pass image path")
= sys.argv[1]
imagePath
= Image.open(imagePath)
im = im.load()
pix = im.size
(w, h)
= [ [ 0 for i in range(int(w / 2)) ] for j in range(int(h / 2)) ]
new
for x in range(int(w / 2)):
for y in range(int(h / 2)):
= 0
l for i in range(x*2, x*2+2):
for j in range(y*2, y*2+2):
= pix[i, j]
r,g,b # Relative luminence formula:
# https://en.wikipedia.org/wiki/Relative_luminance
+= 0.2126*r + 0.7152*g + 0.0722*b
l
# Average them
= l / 4
l
= ''
star if l < 51:
= '★'
star elif l < 102:
= '✦'
star elif l < 153:
= '✡'
star elif l < 204:
= '✧'
star else:
= '☆'
star
= star
new[y][x]
for line in new:
print(''.join(line))
I then copy/paste the star lines into an HTML
<pre>
element, with a little bit of CSS to remove
whitespace between the letters and lines:
/* I know mixing em and px units is bad, bite me. */
pre {line-height: 0.4em;
letter-spacing: -2.8px;
font-family: monospace;
}
I decided to run the original Ziggy Stardust album cover (home of the song Starman) through this script, and I found something really strange!

Turns into…

Holy Starman, Batman! A face appears out of the smoke, in the top left corner of the image.
In case you don’t believe my code (fair!), you can see it for yourself by uploading the album cover image to a black-and-white threshold tool like https://pinetools.com/threshold-image, with a threshold of around 50.

The face doesn’t show quite as clearly this way – I assume it’s the extra compression I added (the 4:1 pixel ratio) that really makes it pop. But still!
You can kinda see the face in the original image, if you squint. I couldn’t find anybody talking about this online – I assume it’s a coincidence? But if anybody knows otherwise, I’d love to hear from you!